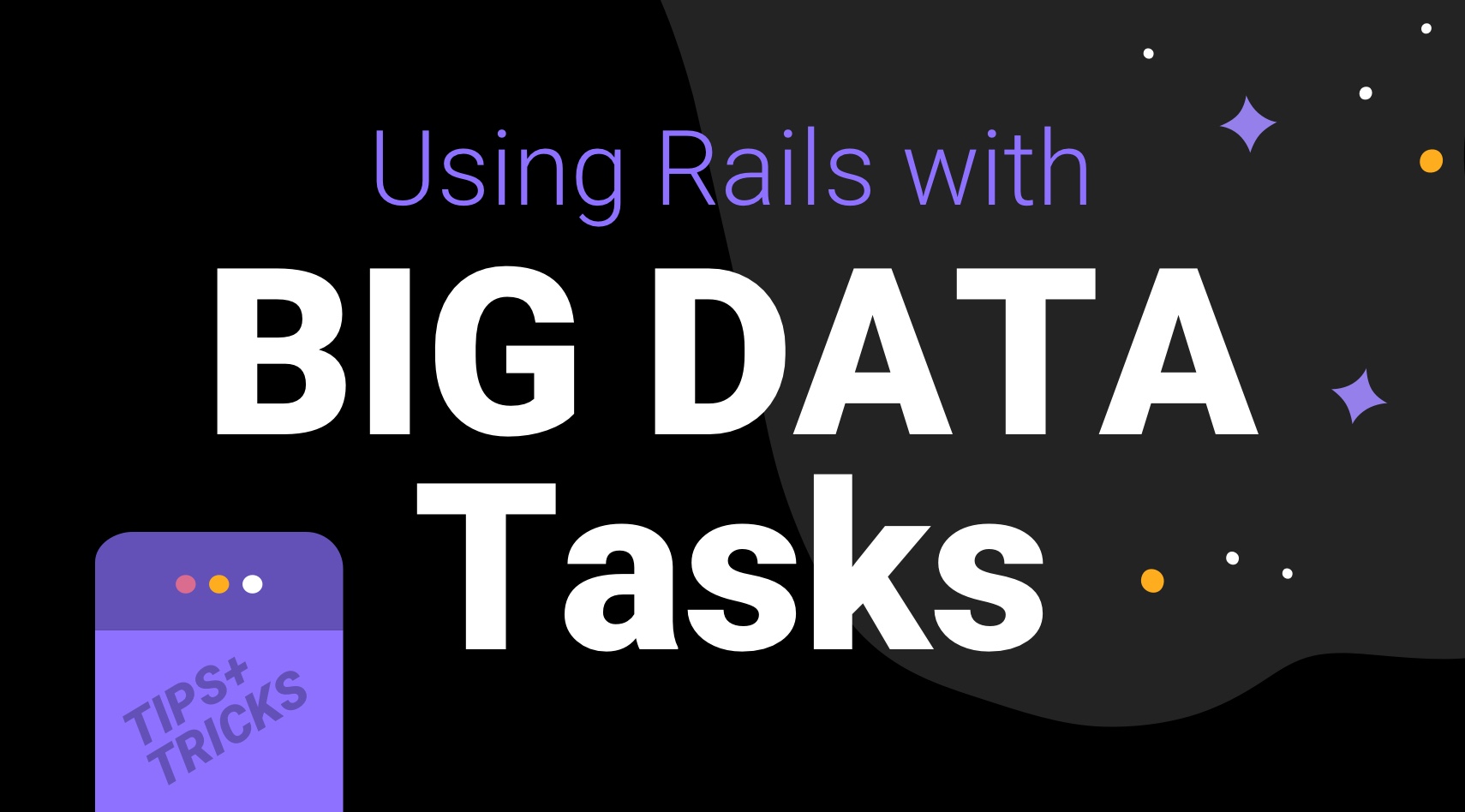
Ruby on Rails: Big Data Tips and Tricks
Big data processing is an essential aspect of modern web applications. Ruby on Rails, a popular web development framework, is often challenged when dealing with millions of records.
However, with the right techniques and optimizations, Rails can handle large datasets efficiently.
Let’s walk through some tips and tricks for managing big data tasks in Ruby on Rails, such as using find_in_batches, adding rescue statements, and more.
1. Use find_each and find_in_batches
When dealing with large datasets, using the regular ActiveRecord methods like “all” or “where” can cause memory issues due to loading all records into memory. Instead, use “find_each” or “find_in_batches,” which fetch records in smaller chunks.
Example:
User.find_each(batch_size: 1000) do |user|
# Process each user record
end
User.find_in_batches(batch_size: 1000) do |users|
users.each do |user|
# Process each user record
end
end
2. Add frequent rescue statements, especially inside loops
When processing a large number of records, a single error shouldn't halt the entire process. To handle exceptions gracefully, add rescue statements inside loops.
Example:User.find_each do |user|
begin
# Process each user record
rescue => e
Rails.logger.error "Error processing user #{user.id}: #{e.message}"
end
end
3. Use pluck or select
When you only need specific attributes from records, avoid loading the entire ActiveRecord object into memory. Use pluck or select to fetch the required columns.
Example:user_emails = User.where(active: true).pluck(:email)
User.where(active: true).select(:id, :email).find_each do |user|
# Process user id and email
end
4. Optimize database queries
Optimize your queries using “includes,” “joins,” or “eager_load” to avoid the N+1 query problem and to ensure efficient use of database resources.
Example:# Using includes
Post.includes(:comments).find_each do |post|
# Process post with preloaded comments
end
# Using joins and select
User.joins(:profile).select('users.*, profiles.name AS profile_name').find_each do |user|
# Process user with profile name
end
# Using eager_load
Post.eager_load(:comments).find_each do |post|
# Process post with preloaded comments
end
5. Use ActiveRecord's update_all and delete_all
When you need to update or delete multiple records with the same conditions, use the “update_all” and “delete_all” methods, which execute a single SQL query.
Example:
# Update all users with the same role
User.where(role: 'guest').update_all(role: 'member')
# Delete all inactive users
User.where(active: false).delete_all
6. Leverage background jobs
For time-consuming tasks or tasks that can be executed asynchronously, use background jobs like Sidekiq, Resque, or Delayed Job. This offloads the work to a separate process and frees up the application server to handle more requests.
Example:Class ProcessUserJob < ActiveJob::Base
queue_as :default
def perform(user_id)
user = User.find(user_id)
# Process the user record
end
end
User.find_each do |user|
ProcessUserJob.perform_later(user.id)
end
7. Monitor performance with an APM Service like Datadog
APM tools like Datadog offer a comprehensive platform for monitoring and analyzing your Rails application to detect where you’re app might be experiencing performance issues or crashing entirely from handling too much data.
Datadog provides several advantages, such as:
- Real-time performance monitoring: Keep an eye on slow database queries, memory usage, and response times to identify areas for optimization.
- Customizable dashboards: Create custom dashboards to visualize and track key performance metrics, making it easier to spot trends and issues.
- APM integration: Datadog's Application Performance Monitoring (APM) offers detailed insights into your application's performance, allowing you to trace individual requests and identify bottlenecks.
- Infrastructure monitoring: Monitor the health of your entire infrastructure, including servers, databases, and other services to ensure a holistic view of your application ecosystem.
- Alerting and anomaly detection: Set up alerts based on predefined or custom thresholds, and leverage Datadog's anomaly detection to identify unusual behavior in your application.
- Collaboration features: Share dashboards, graphs, and alerts with your team to collaborate on identifying and resolving performance issues.
What’s Next
Handling big data tasks in Ruby on Rails can be challenging, but with the right techniques, it is possible to manage large datasets efficiently.
By using methods like find_in_batches, adding rescue statements, optimizing database queries, and leveraging background jobs, you can improve your application's performance while dealing with millions of records.
Keep monitoring and optimizing your code to ensure your application remains performant and reliable.
And if you need guidance on working with technical debt or Rails specifically, see if NextLink Labs’s Custom Software Development service can help you get on track.
Further Reading: How To Build Rails JSON API Serializer